编译常量、ClassLoader类、系统类加载器深度探析
类的初始化
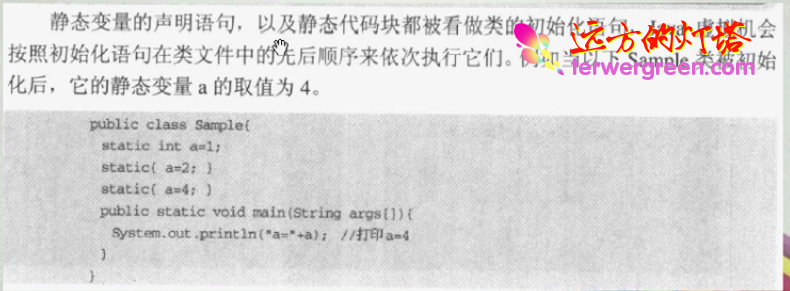

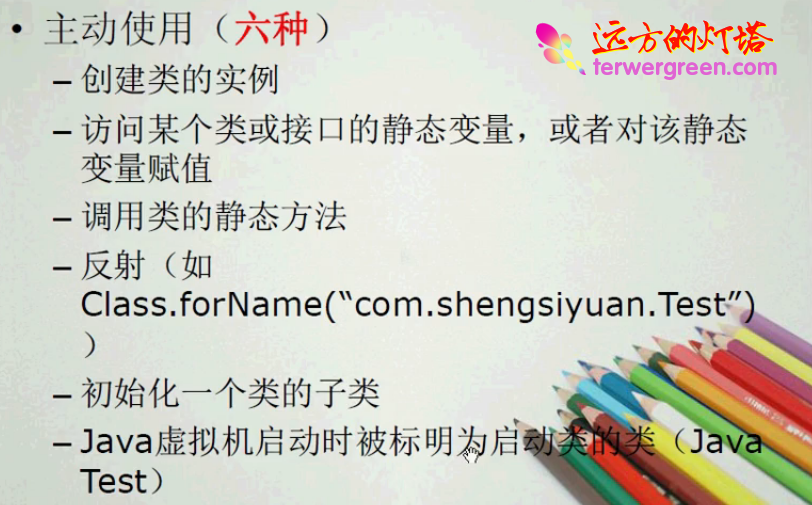
例子:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
|
public class Test2 { public static void main(String[] args) { System.out.println(FinalTest.x); } }
class FinalTest { public static final int x = 6 / 3;
static { System.out.println("FinalTest static block"); } }
|
运行结果

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
|
public class Test3 { public static void main(String[] args) { System.out.println(FinalTest2.x); } }
class FinalTest2{ public static final int x = new Random().nextInt(100);
static { System.out.println("FinalTest2 static block"); } }
|

前面一个 x 是常量,编译时候可以确定,因此,不会初始化类。
后面一个 x 在编译阶段无法确定,运行阶段才能确定因此,会初始化类。
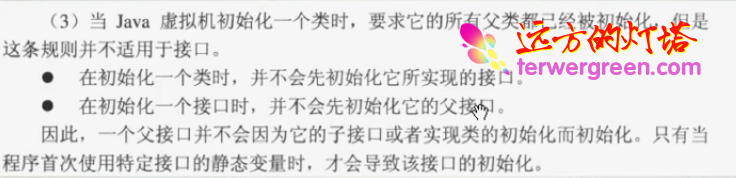
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
|
public class Test4 { static { System.out.println("Test4 static block"); }
public static void main(String[] args) { System.out.println(Child.b); } }
class Parent { static int a = 3;
static { System.out.println("Parent static block"); } }
class Child extends Parent { static int b = 4;
static { System.out.println("Child static block"); } }
|
运行结果
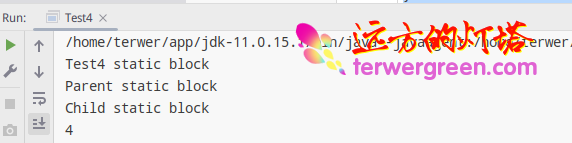
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
|
public class Test5 { static { System.out.println("Test5 static block"); }
public static void main(String[] args) { Parent2 parent;
System.out.println("-----------------");
parent = new Parent2();
System.out.println(Parent2.a);
System.out.println(Child2.b); } }
|
运行结果
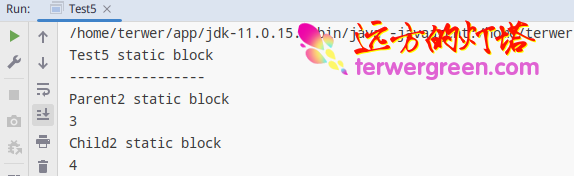
程序中对子类的“主动使用”会导致父类被初始化,但是,对父类的主动使用并不会导致子类初始化(不可能说生成一个 Object 类的对象就导致系统中所有的子类都被初始化)。
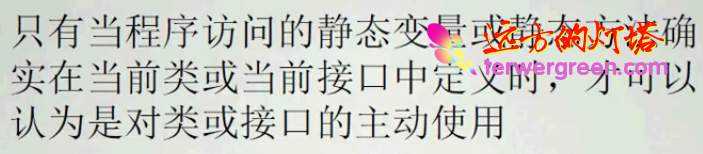
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
public class Test6 { public static void main(String[] args) { System.out.println(Child3.a);
Child3.dosomething(); } }
class Parent3 { static int a = 3;
static { System.out.println("Parent3 static block"); }
static void dosomething() { System.out.println("do something"); } }
class Child3 extends Parent3 { static { System.out.println("Child3 static block"); } }
|
运行结果
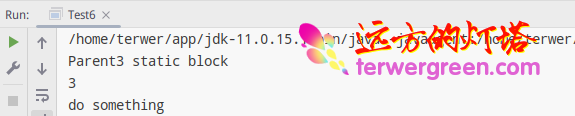
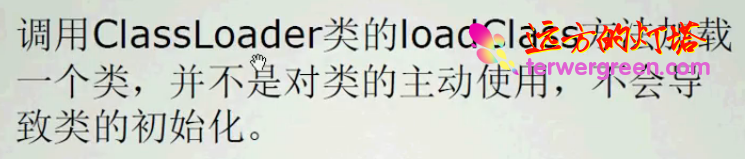
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
public class Test7 { public static void main(String[] args) throws Exception { ClassLoader classLoader = ClassLoader.getSystemClassLoader(); Class<?> clazz = classLoader.loadClass("com.terwergreen.classloader.C"); System.out.println("-------------------");
Class.forName("com.terwergreen.classloader.C"); } }
class C { static { System.out.println("Class C"); } }
|
运行效果
